Understanding Synchronous and Asynchronous Callback Functions: A Comprehensive Guide
In modern programming, especially in JavaScript, handling tasks that might take some time (like fetching data from a server or reading a file) is crucial. To manage these tasks efficiently, developers use callback functions. Callbacks can be synchronous or asynchronous, each serving different purposes. This article will break down these concepts, provide real-world examples, and discuss the notorious “callback hell” as it involves Javascript
1. What is a Callback Function?
A callback function is a function that is passed as an argument to another function and is executed after the first function has completed its operation. The idea is to ensure that certain code is run only after some other code has finished executing.
Callbacks are common in JavaScript due to its single-threaded, non-blocking nature. The event-driven architecture of JavaScript makes callbacks essential for handling operations that are expected to take time.
2. Synchronous Callback Functions
A synchronous callback is executed immediately after the function it’s passed into is completed. These callbacks block the execution of subsequent code until they have completed.
Example of a Synchronous Callback
function greet(name) {
console.log(‘Hello ‘ + name);
}
function getUserInput(callback) {
const name = prompt(‘Please enter your name:’);
callback(name);
}
// Calling getUserInput with greet as the callback function
getUserInput(greet);
In this example:
- greet is a synchronous callback function that is called immediately after getUserInput has finished getting the user input.
- The execution of getUserInput is paused until the prompt function is resolved (i.e., the user enters their name).
Pros of Synchronous Callbacks:
- Simple and easy to understand.
- Execution order is straightforward and predictable.
Cons of Synchronous Callbacks:
- Blocks the execution of other code until it completes, which can lead to a poor user experience (e.g., a frozen UI).
3. Asynchronous Callback Functions
An asynchronous callback is executed after the completion of some asynchronous operation. It doesn’t block the execution of subsequent code, allowing the program to continue running while waiting for the operation to complete.
Example of an Asynchronous Callback
console.log(‘Start’);
setTimeout(() => {
console.log(‘This is an asynchronous callback’);
}, 2000);
console.log(‘End’);
In this example:
- setTimeout is an asynchronous function that takes a callback function and a delay time in milliseconds.
- The callback function is executed after a 2-second delay, allowing the code to continue running.
The output will be:
sql
Copy code
Start
End
This is an asynchronous callback
Pros of Asynchronous Callbacks:
- Non-blocking: They allow other code to run while waiting.
- Useful for operations like fetching data, file handling, or interacting with APIs.
Cons of Asynchronous Callbacks:
- Can be harder to reason about due to the non-linear flow of execution.
- Potentially leads to callback hell if not managed properly.
4. Understanding Callback Hell
Callback Hell refers to a situation where multiple asynchronous operations are nested within one another, leading to deeply nested code that is difficult to read and maintain. This often happens when one callback relies on the result of another, leading to a “pyramid of doom.”
Example of Callback Hell
function getData(callback) {
setTimeout(() => {
console.log(‘Fetching data…’);
callback(‘data’);
}, 1000);
}
function processData(data, callback) {
setTimeout(() => {
console.log(‘Processing ‘ + data);
callback(‘processed data’);
}, 1000);
}
function saveData(data, callback) {
setTimeout(() => {
console.log(‘Saving ‘ + data);
callback(‘saved data’);
}, 1000);
}
// Callback hell
getData((data) => {
processData(data, (processedData) => {
saveData(processedData, (savedData) => {
console.log(‘All done: ‘ + savedData);
});
});
});
In this example:
- Each asynchronous function is nested within another, creating a deeply nested structure that is hard to follow.
- As the complexity of the code grows, it becomes increasingly difficult to maintain and debug.
Consequences of Callback Hell:
- Makes the code less readable.
- Increases the risk of bugs.
- Makes it challenging to add or modify features.
5. Avoiding Callback Hell: Promises and Async/Await
To mitigate callback hell, JavaScript introduced Promises and later Async/Await syntax, both of which provide more readable ways to handle asynchronous code.
Example Using Promises
function getData() {
return new Promise((resolve) => {
setTimeout(() => {
console.log(‘Fetching data…’);
resolve(‘data’);
}, 1000);
});
}
function processData(data) {
return new Promise((resolve) => {
setTimeout(() => {
console.log(‘Processing ‘ + data);
resolve(‘processed data’);
}, 1000);
});
}
function saveData(data) {
return new Promise((resolve) => {
setTimeout(() => {
console.log(‘Saving ‘ + data);
resolve(‘saved data’);
}, 1000);
});
}
// Using Promises to avoid callback hell
getData()
.then(processData)
.then(saveData)
.then((savedData) => {
console.log(‘All done: ‘ + savedData);
})
.catch((error) => {
console.error(‘Error:’, error);
});
Example Using Async/Await
async function handleData() {
try {
const data = await getData();
const processedData = await processData(data);
const savedData = await saveData(processedData);
console.log(‘All done: ‘ + savedData);
} catch (error) {
console.error(‘Error:’, error);
}
}
handleData();
With Promises and Async / Await:
- The code is more readable and easier to maintain.
- The linear flow is restored, making it easier to follow the logic.
- Error handling is simpler using .catch() for Promises or try/catch for Async/Await.
Conclusion
Synchronous and asynchronous callbacks are foundational concepts in JavaScript, crucial for managing operations that take time. While synchronous callbacks are simpler and straightforward, they can block the execution of other code. Asynchronous callbacks prevent blocking but can lead to complex, hard-to-maintain code structures like callback hell.
Using modern JavaScript features like Promises and Async/Await, developers can avoid callback hell, making their code cleaner, more readable, and easier to maintain. Understanding when and how to use these tools is vital for writing efficient, effective JavaScript code. For more of this you can contact Nanocodes programming limited@www.nanocodes.com.ng
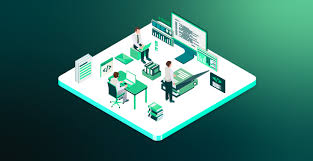